Being able to preview your transition animations right in Xcode will allow you to quickly make changes to get the perfect effect you're looking for.
While updating SwiftUI Animations Mastery for iOS 15, I decided to take a deeper look into previewing transition animations.
Some work. Some do not.
Sometimes the preview works depending on how you write your animation.
Let's look at some examples.
What We Want
This is what we want to see:
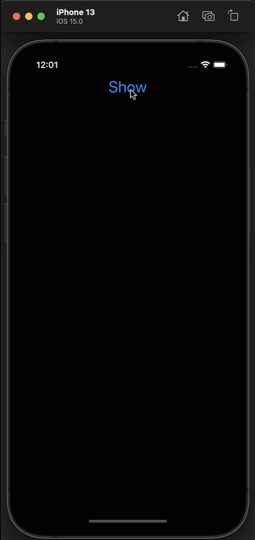
Pretty simple. An image slides in and out.
Animation on Transition Type
Adding an animation directly to a transition no longer works.
Example:
if show {
Image("driving")
.transition(AnyTransition.slide.animation(.default))
}
Does it work?
- Preview - No
- Simulator - No
- Device - No
Animation Modifier
Using an animation modifier on what you are showing/hiding no longer works.
Example:
if show {
Image("driving")
.transition(AnyTransition.slide)
.animation(.default, value: show)
}
Does it work?
- Preview - No
- Simulator - No
- Device - No
Using withAnimation
When using withAnimation it partially works.
Example:
Button("Show") {
withAnimation {
show.toggle()
}
}
.font(.title)
Spacer()
if show {
Image("driving")
.transition(AnyTransition.slide)
}
Does it work?
- Preview - No
- Simulator - Yes
- Device - Yes
How to get withAnimation to work with Preview?
The withAnimation seems like a good solution, it's just not working with the Preview at the time of this writing. (I have filed a Feedback on this.)
The good news is, there IS a workaround.
In your PreviewProvider, wrap your view in a VStack.
Example:
struct MyView_Previews: PreviewProvider {
static var previews: some View {
VStack { // Workaround
PreviewingSwiftUITransitionAnimations_Blog()
}
}
}
Does it work?
- Preview - Yes
- Simulator - Yes
- Device - Yes
The Preview will now correctly show the transition animation.
I do not know why this works. If you do, leave a comment and let us know.
I added it to the book:
Using Group
If you cannot use withAnimation for some reason, you can wrap your if statement in a Group.
Example:
Group {
if show {
Image("driving")
.transition(AnyTransition.slide)
}
}
.animation(.default, value: show)
Does it work?
- Preview - Yes
- Simulator - Yes
- Device - Yes
Note: You do not need to embed your view in a VStack in your PreviewProvider to make this work.
Summary
To be able to preview your transition animations, use one of the following:
- Use withAnimation and embed your view in a VStack inside the PreviewProvider.
- Embed your if condition within a Group (or any other container) and add the animation to the container.
Robert McGovern